Calibration of non-linear (logarithmic) function#
from pylab import *
%pylab inline
from io import StringIO
%pylab is deprecated, use %matplotlib inline and import the required libraries.
Populating the interactive namespace from numpy and matplotlib
/home/user/mambaforge/envs/mdd/lib/python3.10/site-packages/IPython/core/magics/pylab.py:162: UserWarning: pylab import has clobbered these variables: ['random', 'fft', 'power']
`%matplotlib` prevents importing * from pylab and numpy
warn("pylab import has clobbered these variables: %s" % clobbered +
# create two signals: concentration and temperature
c = StringIO("""
1.095406121 3.887032952 6.956500526 9.486921797 \
13.96944459 14.86018043 23.19810833 24.53008787 \
24.72311112 37.44113657 38.05523491 54.1881169""")
T = StringIO("""91.72763561 70.60278306 \
53.0039356 45.03419592 32.45847839 29.03763728 13.49252686 \
12.0641877 18.91647307 12.01351046 11.49379565 9.671537342 """)
c = loadtxt(c)
T = loadtxt(T)
plot(c,T,'o')
[<matplotlib.lines.Line2D at 0x7f377259f820>]
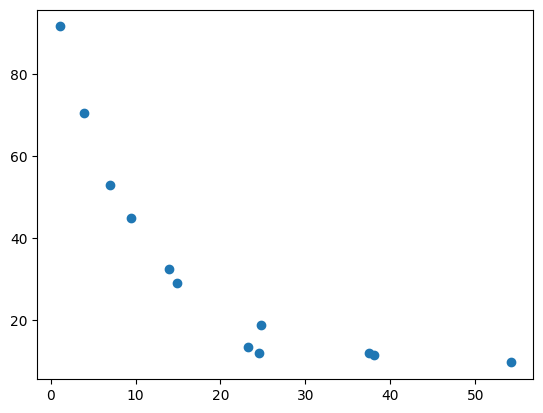
a = -np.log10(T)
plot(c,a,'o')
[<matplotlib.lines.Line2D at 0x7f3772444e50>]
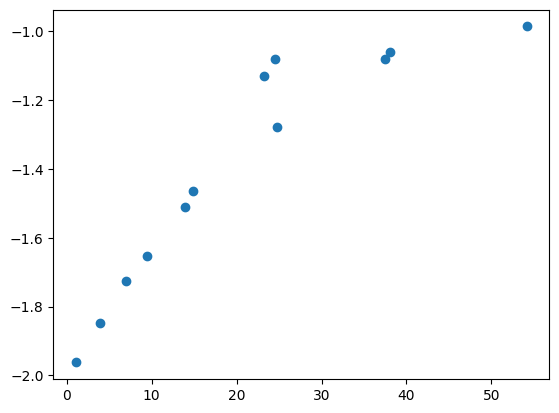
see the linear part and the “saturated part”, use only the linear one#
ind = c < 24
plot(c[ind],a[ind],':o')
[<matplotlib.lines.Line2D at 0x7f37702ae1d0>]
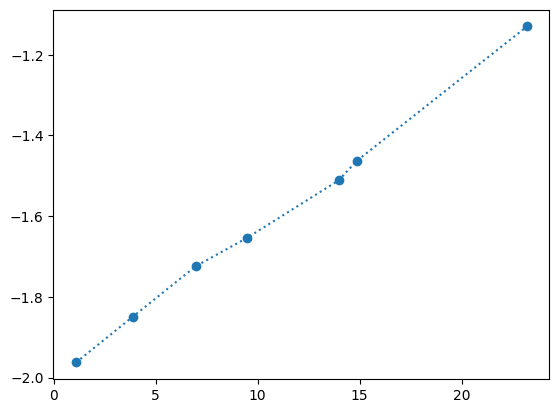
polyfit(c[ind],a[ind],1)
array([ 0.03674248, -1.99891754])
plot(c,a,'o')
c1 = linspace(0,60,100)
a1 = 0.037*c1-2.0
plot(c1,a1,'--')
[<matplotlib.lines.Line2D at 0x7f377031a6e0>]
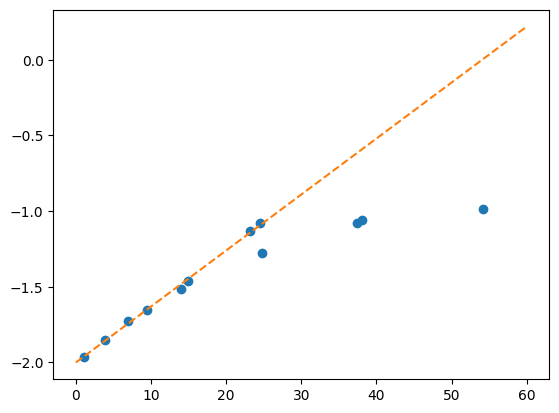
plot(c,T,'o')
a1 = 0.037*c1-2.0
plot(c1,10**(-a1),'--')
[<matplotlib.lines.Line2D at 0x7f377018b6a0>]
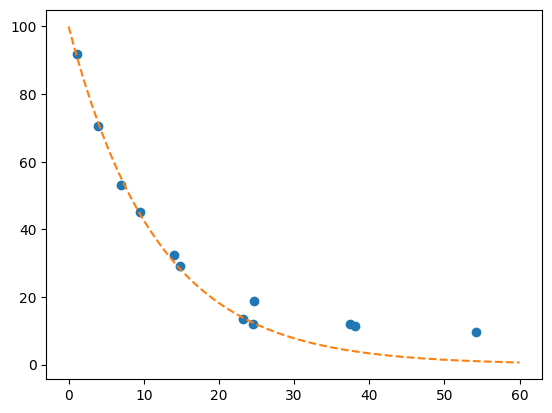
print(f'c = {c}')
print(f'T = {T}')
c = [ 1.09540612 3.88703295 6.95650053 9.4869218 13.96944459 14.86018043
23.19810833 24.53008787 24.72311112 37.44113657 38.05523491 54.1881169 ]
T = [91.72763561 70.60278306 53.0039356 45.03419592 32.45847839 29.03763728
13.49252686 12.0641877 18.91647307 12.01351046 11.49379565 9.67153734]
plot(c,T,'bo',c[6:8],T[6:8],'rs')
[<matplotlib.lines.Line2D at 0x7f3770221570>,
<matplotlib.lines.Line2D at 0x7f3770221600>]
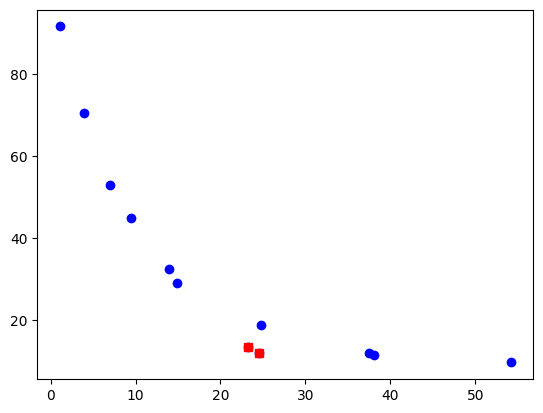
c2 = c.copy()
T2 = T.copy()
mask = ones(c2.shape[0],dtype=bool)
mask[[6,7]] = False
plot(c2[mask],T2[mask],'o')
[<matplotlib.lines.Line2D at 0x7f3770099390>]
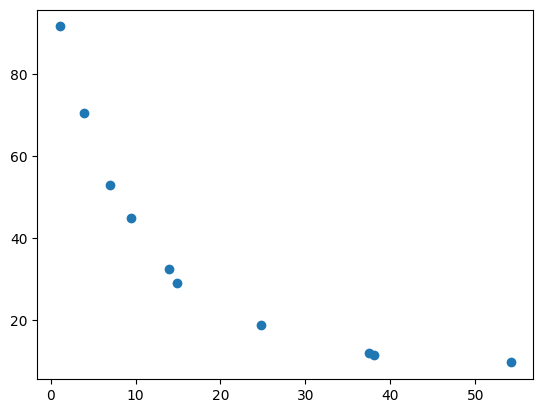
plot(c2[mask]-c2[0],T2[mask]-T2[0],'o')
[<matplotlib.lines.Line2D at 0x7f3770100f10>]
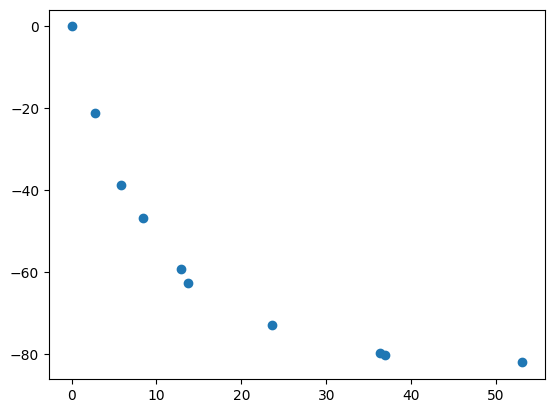
c3 = c2[mask] - c2[0]
T3 = T2[0] - T2[mask]
c3
array([ 0. , 2.79162683, 5.86109441, 8.39151568, 12.87403847,
13.76477431, 23.627705 , 36.34573045, 36.95982879, 53.09271078])
loglog(c3,T3,'o')
[<matplotlib.lines.Line2D at 0x7f3770175030>]
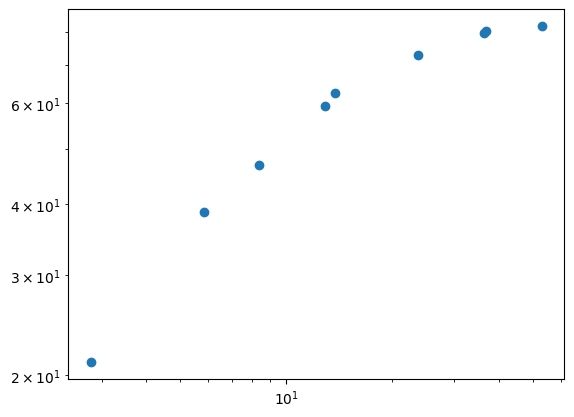